Domain Modeling
Do not model the current state of the world, model how you get there
event sourcing by example
Discover
We can illustrate a system’s expected behavior from start to finish on a timeline, without branching, using a concrete example.
Event Modeling is a powerful technique for exploring a domain. It describes systems by tracing how information changes over time through key events. These events, placed on a timeline, provide a clear and structured representation of the system.
Learn more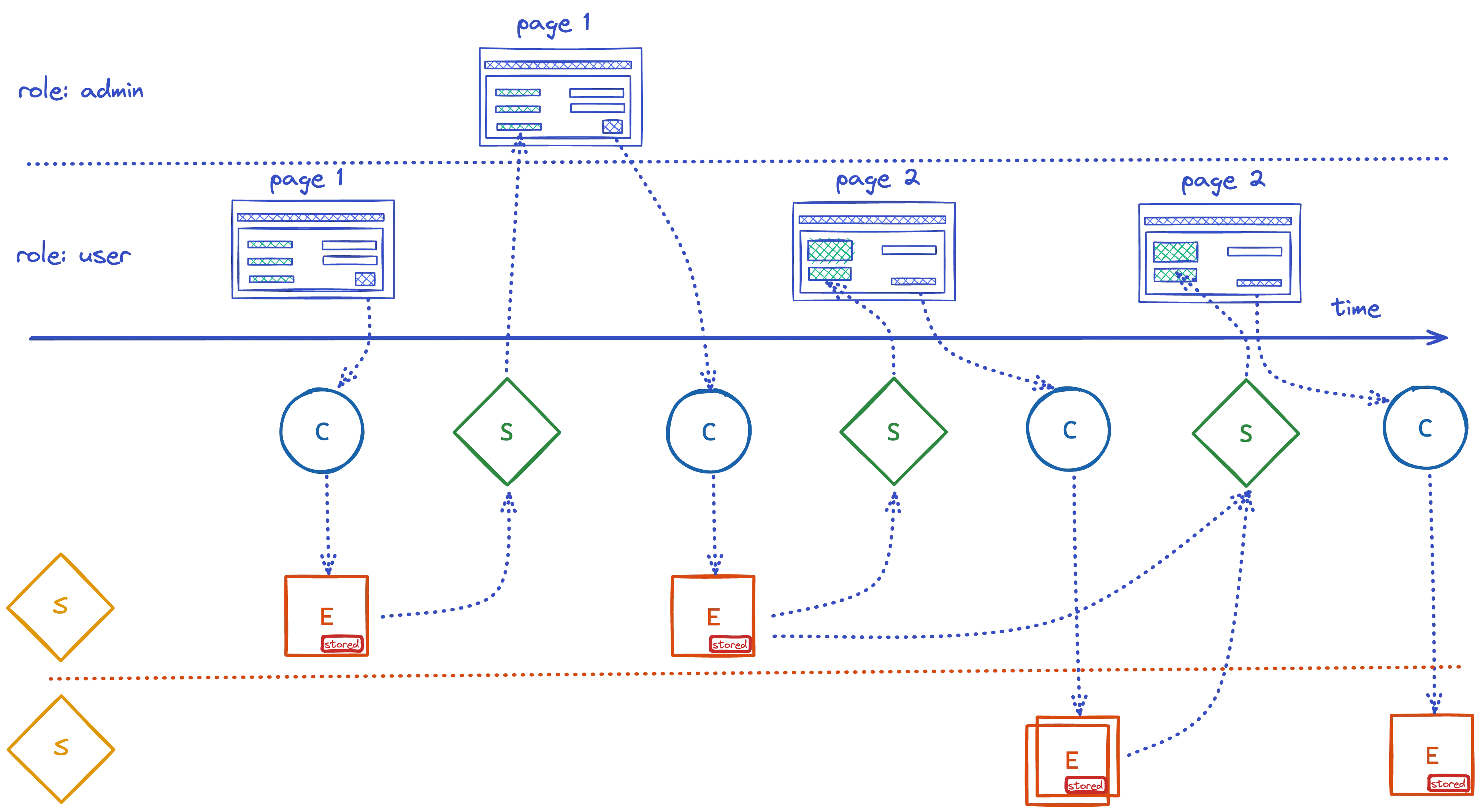
Distill
Requirements are expressed as scenarios.
A scenario represents the system's behavior from the user's perspective and follows the Given-When-Then structure to create a testable specification.
The specification is written as executable tests, which are automated and run continuously. These tests cover a full range of scenarios, from happy paths to error cases.
Learn more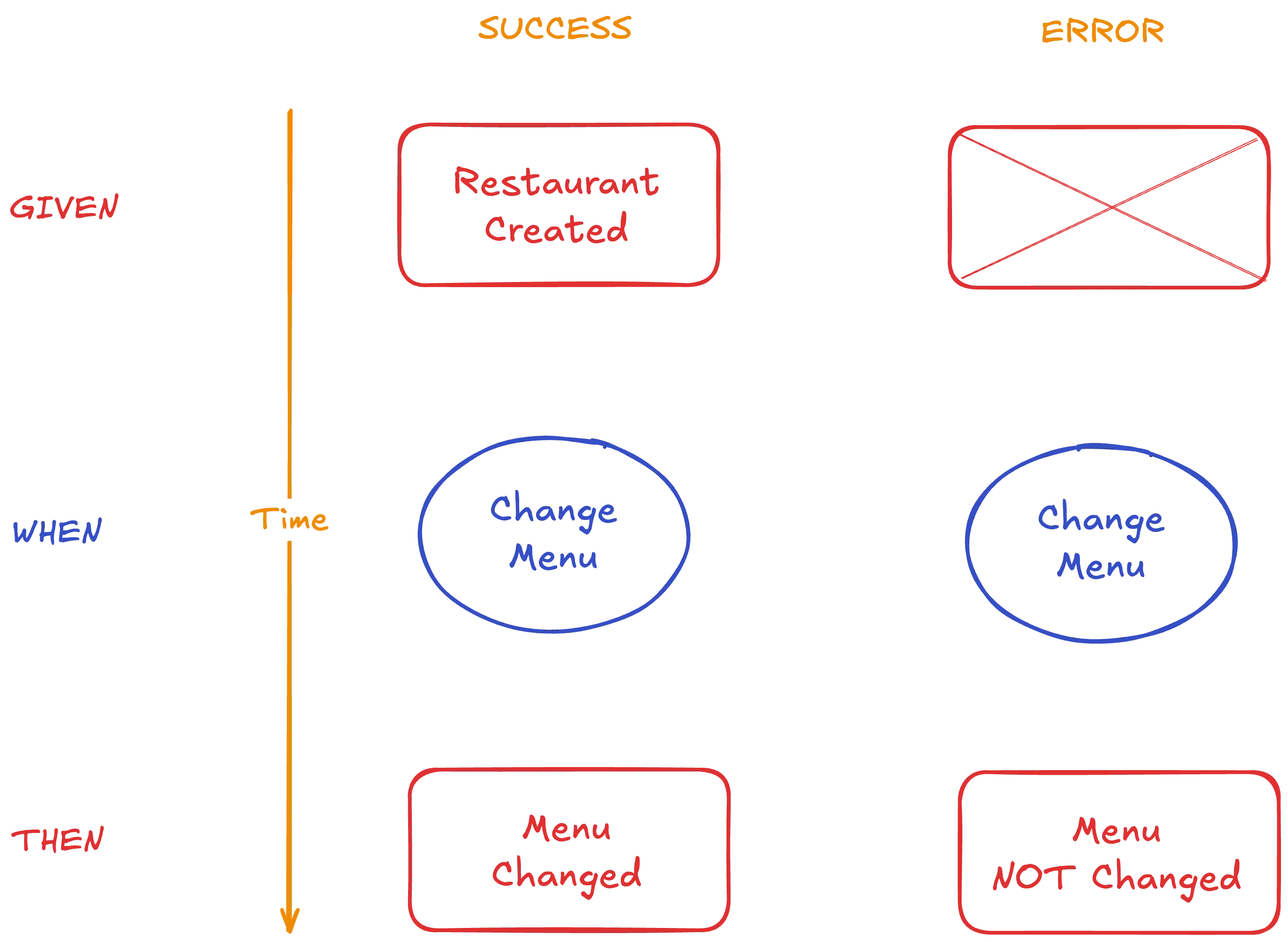
Deno.test(function changeRestaurantMenuDeciderTest() {
const changeRestaurantMenuCommand: RestaurantCommand = restaurantCommandSchema
.parse(
JSON.parse(changeRestaurantMenuCommandJson),
);
const restaurantCreatedEvent: RestaurantEvent = restaurantEventSchema
.parse(
JSON.parse(restaurantCreatedEventJson),
);
const restaurantMenuChangedEvent: RestaurantEvent = restaurantEventSchema
.parse(
JSON.parse(restaurantMenuChangedEventJson),
);
DeciderSpecification.for(restaurantDecider)
.given([restaurantCreatedEvent])
.when(changeRestaurantMenuCommand)
.then([restaurantMenuChangedEvent]);
});
Develop
The f { model }
library is implementing the event model in a general way. It promotes clear separation between data and behaviour.
The flow is parametrized with Command, Event, and State parameters. The responsibility of the business is to specialize in their case by specifying concrete Commands, Events, and State. For example, Commands=CreateOrder, AddItemToOrder
; Events=OrderCreated, ItemAdded;
State=Order
(with list of Items).
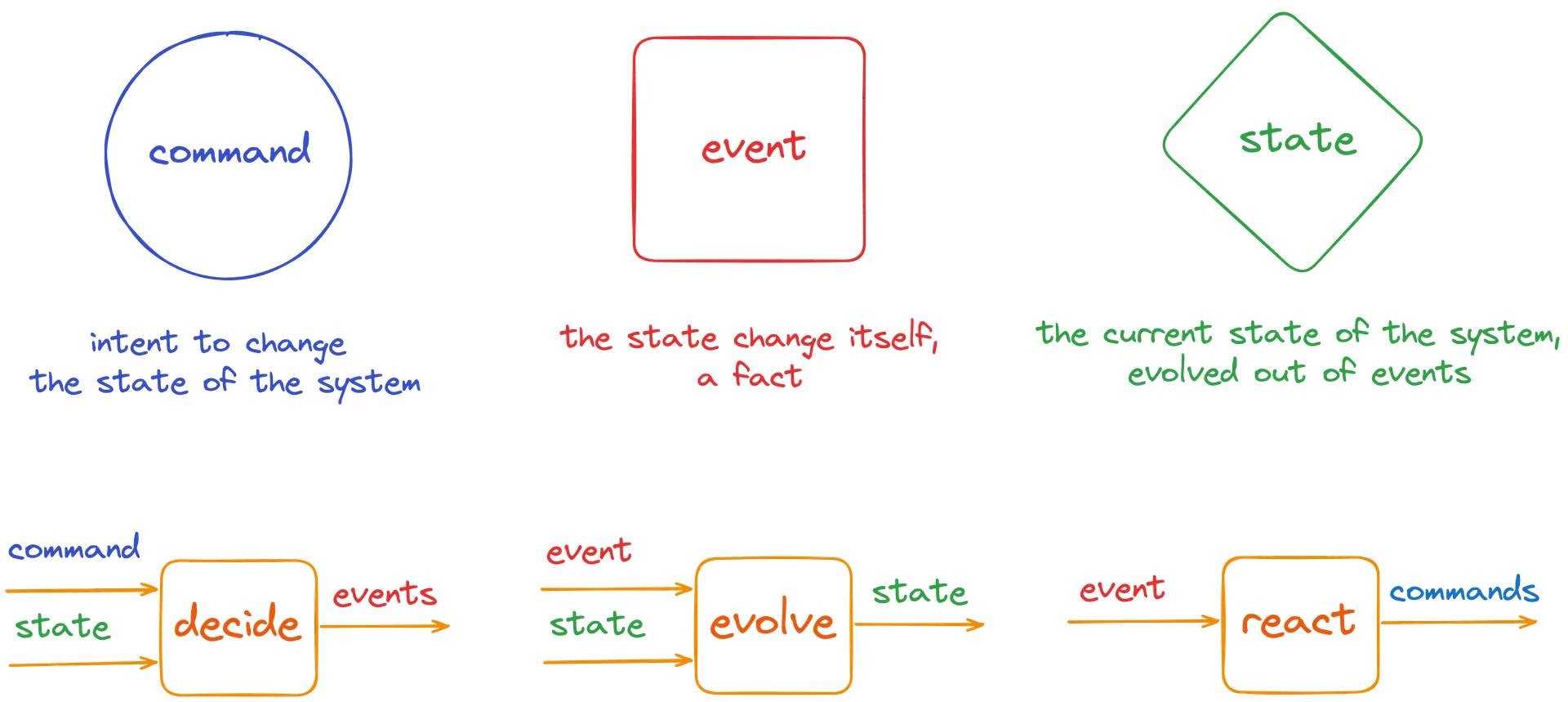
// Restaurant Commands
export type RestaurantCommand =
| CreateRestaurantCommand
| ChangeRestaurantMenuCommand
| PlaceOrderCommand;
export type CreateRestaurantCommand = {
readonly decider: "Restaurant";
readonly kind: "CreateRestaurantCommand";
readonly id: RestaurantId;
readonly name: RestaurantName;
readonly menu: RestaurantMenu;
};
export type ChangeRestaurantMenuCommand = {
readonly decider: "Restaurant";
readonly kind: "ChangeRestaurantMenuCommand";
readonly id: RestaurantId;
readonly menu: RestaurantMenu;
};
// A Restaurant Decider
export const restaurantDecider: Decider<
RestaurantCommand,
Restaurant | null,
RestaurantEvent
> = new Decider<RestaurantCommand, Restaurant | null, RestaurantEvent>(
(command, currentState) => {
// Ehaustive switch/pattern matching
switch (command.kind) {
case "CreateRestaurantCommand":
...
Database
event-sourcing
and event-streaming
with PostgreSQL database.
Optimistic Locking
- for concurrency controlConcurrent Consumers
- for efficient streamingAt-Least-Once Delivery
- to ensure reliability
No additional tools, frameworks, or programming languages are required at this level.
Learn more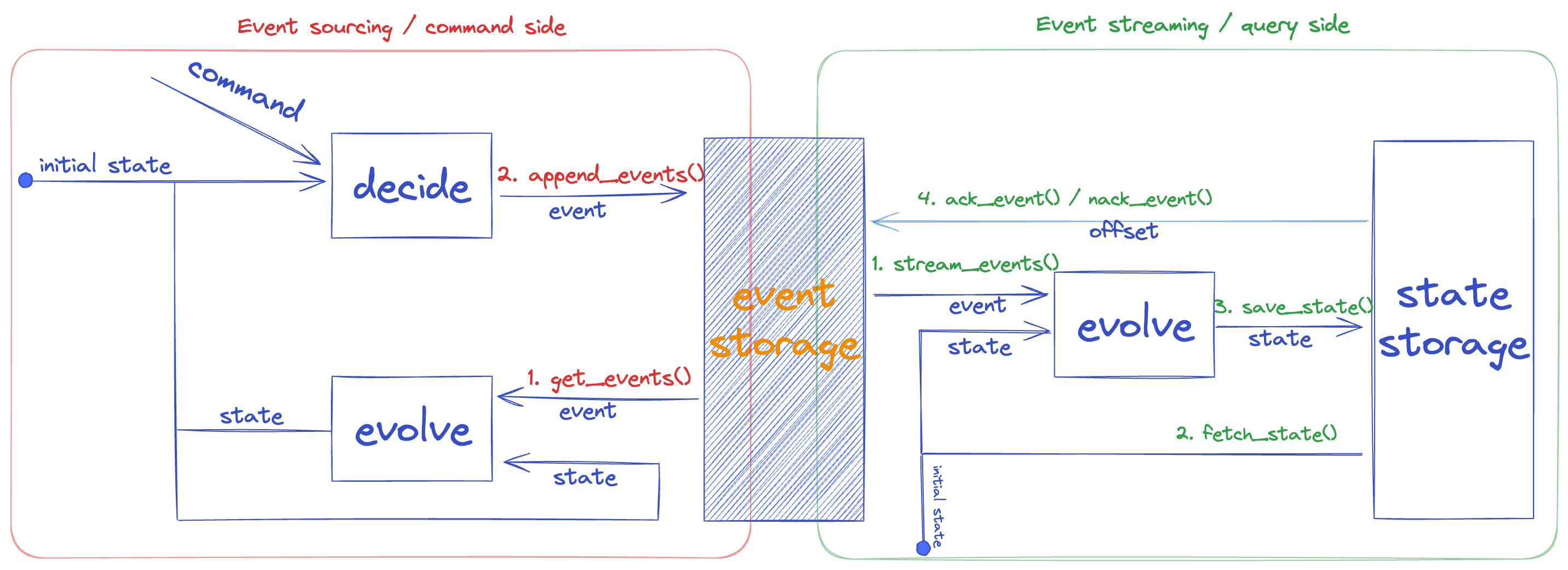
CREATE TABLE IF NOT EXISTS events
(
-- event name/type. Part of a composite foreign key to deciders
"event" TEXT NOT NULL,
-- event ID. This value is used by the next event as its previous_id value to implement optimistic locking effectively.
"event_id" UUID NOT NULL UNIQUE,
-- event version. This value represents the version of the event schema.
"event_version" BIGINT NOT NULL DEFAULT 1,
-- decider name/type. Part of a composite foreign key to deciders
"decider" TEXT NOT NULL,
-- identifier for the decider
"decider_id" TEXT NOT NULL,
-- event data in JSON format
"data" JSONB NOT NULL,
-- command ID causing this event
"command_id" UUID NOT NULL,
-- previous event uuid; null for first event; null does not trigger UNIQUE constraint; we defined a function check_first_event_for_decider
"previous_id" UUID UNIQUE,
-- indicator if the event stream for the decider_id is final
"final" BOOLEAN NOT NULL DEFAULT FALSE,
-- The timestamp of the event insertion.
"created_at" TIMESTAMP WITH TIME ZONE DEFAULT NOW() NOT NULL,
-- ordering sequence/offset for all events, in all deciders.
"offset" BIGSERIAL PRIMARY KEY,
-- postgres transaction id
"transaction_id" XID8 DEFAULT pg_current_xact_id() NOT NULL,
FOREIGN KEY ("decider", "event", "event_version") REFERENCES deciders ("decider", "event", "event_version")
);
CREATE OR REPLACE FUNCTION append_event(v_event TEXT, v_event_id UUID, v_decider TEXT, v_decider_id TEXT, v_data JSONB,
v_command_id UUID, v_previous_id UUID, v_event_version BIGINT DEFAULT 1)
RETURNS SETOF events AS
'
INSERT INTO events (event, event_id, event_version, decider, decider_id, data, command_id, previous_id)
VALUES (v_event, v_event_id, v_event_version, v_decider, v_decider_id, v_data, v_command_id, v_previous_id)
RETURNING *;
' LANGUAGE sql;